Oct 5, 2024
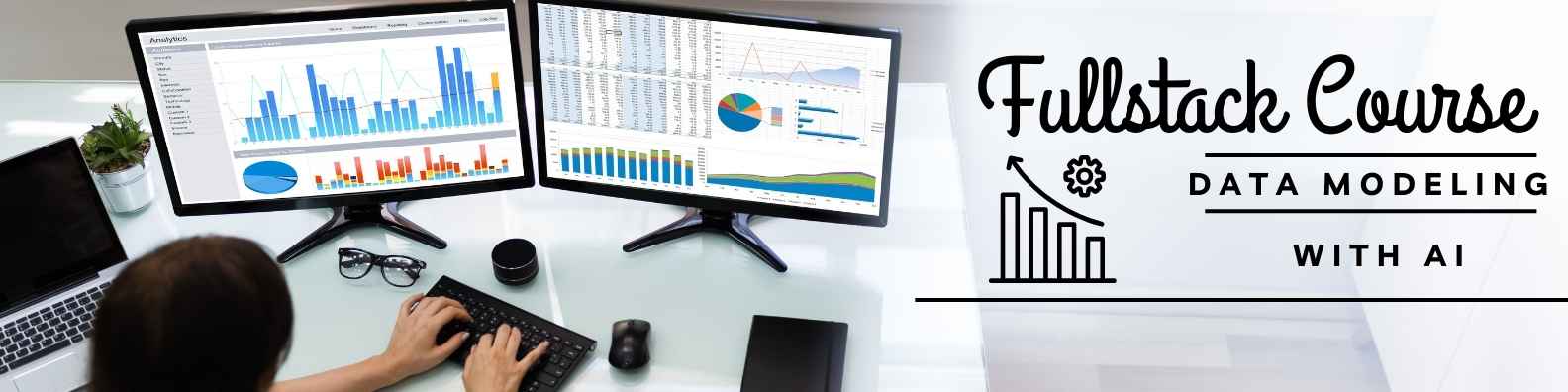
Fullstack Course: Chapter 2 - Data Modeling using AI Prompts
As a fullstack developer, mastering data modeling is crucial for designing efficient, scalable applications. Data modeling forms the backbone of both your backend and frontend logic, influencing database structure, API design, and even the way your UI interacts with data. In this chapter, we’ll explore key concepts in data modeling, best practices, and how to apply them in a fullstack environment.
1. What is Data Modeling?
Data modeling is the process of defining the structure, relationships, and constraints of data used in an application. It involves creating abstract representations (models) of the data and defining how different data entities relate to each other. This often translates into a database schema for relational databases or collections and documents in NoSQL systems.
2. Types of Data Models
Data models can be categorized into three primary types:
- Conceptual Data Model: This is a high-level representation of business entities and their relationships. It is technology-agnostic and focuses on what data is important for the business, rather than how it is stored.
- Logical Data Model: This dives deeper into the specifics, laying out data attributes and their relationships in detail. It's still technology-agnostic but includes more concrete decisions, such as data types (e.g., integer, string).
- Physical Data Model: This is the implementation-level model, where the actual database design takes place. Here, decisions like indexing, normalization, and constraints (e.g., foreign keys, primary keys) are made, and the data types are tied to specific DBMS capabilities.
3. Key Concepts in Data Modeling
Before diving into specific implementations, it's important to understand the following concepts:
3.1 Entities and Attributes
- Entity: A real-world object or concept. For example, in an e-commerce app, entities might include User, Product, and Order.
- Attribute: A property of an entity. For a User entity, attributes could include username, email, password, and date_of_birth.
3.2 Relationships
Entities often relate to one another. There are three primary types of relationships:
- One-to-One (1:1): Each entity in the relationship corresponds to exactly one entity in the related table. E.g., a User can have exactly one Profile.
- One-to-Many (1:N): One entity in the relationship corresponds to multiple entities in the related table. E.g., a User can have multiple Orders.
- Many-to-Many (N:N): Both entities can have multiple related entities. E.g., Products and Categories in an e-commerce app.
3.3 Normalization vs. Denormalization
- Normalization: The process of organizing a database to reduce redundancy and improve integrity by dividing data into related tables. For example, separating user addresses into a different table linked to the user table by a foreign key.
- Denormalization: Combining related tables into fewer, larger tables to reduce the number of joins and speed up read performance. This is often used in NoSQL databases or for performance optimization in relational systems.
3.4 Primary Keys and Foreign Keys
- Primary Key (PK): A unique identifier for a record in a table. It must be unique and not null. E.g., user_id for a User table.
- Foreign Key (FK): A field in one table that uniquely identifies a row in another table, establishing a relationship between the two tables.
3.5 Indexes
Indexes are used to improve the speed of data retrieval. However, over-indexing can degrade performance during data insertions, updates, or deletions. Choose indexes carefully, prioritizing fields that are commonly used in search queries or joins.
4. Relational vs. NoSQL Databases
As a fullstack developer, you’ll likely work with both relational and NoSQL databases, and understanding how to model data for each is crucial.
4.1 Relational Databases
Relational databases like PostgreSQL, MySQL, and SQL Server are designed to store data in a structured, table-based format with well-defined relationships. The data is modeled using normalized schemas and constraints, which help maintain consistency and integrity.
- Example of a relational schema:
CREATE TABLE users ( user_id SERIAL PRIMARY KEY, username VARCHAR(100), email VARCHAR(100) UNIQUE, password_hash TEXT ); CREATE TABLE orders ( order_id SERIAL PRIMARY KEY, user_id INT REFERENCES users(user_id), total_amount DECIMAL, order_date TIMESTAMP );
4.2 NoSQL Databases
NoSQL databases like MongoDB, DynamoDB, and Couchbase are more flexible in storing unstructured or semi-structured data. They are often used in high-traffic applications that require horizontal scaling and can handle large amounts of data with fewer constraints.
- Document-oriented NoSQL Example (MongoDB):
{ "user_id": "abc123", "username": "john_doe", "email": "john@example.com", "orders": [ { "order_id": "o1", "total_amount": 59.99, "order_date": "2024-01-10" }, { "order_id": "o2", "total_amount": 22.50, "order_date": "2024-02-15" } ] }
In NoSQL, denormalization is common, where related entities are embedded into a document to improve query performance by avoiding costly joins.
Old Way Of Data Modeling
5. Data Modeling in Fullstack Development
5.1 Backend Considerations
Your backend, whether Node.js with Express, Django, or any other framework, will need to interact with the database efficiently. When modeling data, think about:
- CRUD operations: Ensure your data model supports create, read, update, and delete (CRUD) operations easily.
- Query performance: Design for efficient data retrieval, especially for common queries. Use appropriate indexes and data structures.
- Consistency and Integrity: For relational databases, use constraints like foreign keys and unique indexes to maintain data integrity.
- Scalability: If your application is expected to scale, design your data model with sharding or partitioning in mind for NoSQL, and consider read-write separation strategies for relational databases.
5.2 Frontend Considerations
On the frontend, data modeling influences how data is structured and manipulated in the client-side application. Consider:
- Data shape: The structure of your API responses should match the data needs of your UI components. Flatten or denormalize data where necessary to reduce frontend complexity.
- State management: How will data be managed on the frontend? Ensure the data model supports efficient state updates, whether using tools like Redux, Zustand, or React’s context API.
- Pagination and Filtering: For large datasets, ensure your API and data model allow for efficient pagination and filtering mechanisms.
5.3 API Design
When designing APIs, data models play a critical role in determining:
- RESTful resource design: Each entity should have a corresponding API resource. Design endpoints that map well to the underlying data model.
- Example:
/api/users/{id}/orders
to fetch orders for a specific user. - GraphQL schema: In a GraphQL API, your data model will influence the types, queries, and mutations you define.
- Example:
type User { id: ID! username: String! orders: [Order] }
6. Best Practices in Data Modeling
- Start simple: Avoid over-complicating your data model. Begin with simple relationships and grow complexity as needed.
- Model for the use case: Your data model should reflect how your application is used, not just how data is stored. Optimize for common read and write operations.
- Evolve over time: Your data model will evolve as requirements change. Ensure your application can handle schema migrations, whether through database migrations (SQL) or versioning strategies (NoSQL).
- Test and optimize: Continuously test your queries and data structures for performance, especially as data grows. Optimize indexes and refine data models as needed.
New Way Of Data Modeling - Holistic Programming With AI Prompts
7. Using AI Prompts for Data Modeling
The traditional data modeling practices explained in step 1 to 6 can take months to complete for a mid-size software and there may be regular optimization requirement. But use of AI prompts can speed up and automate the design, development and maintenance of data models.
The following prompt sets are used for generating the data model for this type of NodejS ExpressJS MVCC stack.
Here is the initiation code for automating the Sequelize based modeling:
const Sequelize = require("sequelize"); const sequelize = require("../../config/database"); const { v4: uuidv4 } = require("uuid"); const tableName = "@Function"; const @Function = sequelize.define( "@Function", { id: { // Unique ID type: Sequelize.STRING, defaultValue: Sequelize.UUIDV4, primaryKey: true, }, }, { indexes: [], createdAt: "cdt", updatedAt: "ldt", tableName, } ); module.exports = @Function;
The instruction prompt is as follows:
Add following data types in the model with appropriate type, defaultValue and allowNull values, if it doesn't exist already. @Model
That is all you need for automating the design, development and maintenance of data models. Just follow the prompt design principle as explained here.
8. Conclusion
Data modeling is essential for building robust fullstack applications, influencing everything from database design to API structure and frontend state management. Understanding the different types of databases, how to structure entities and relationships, and how to balance between normalization and denormalization will enable you to create scalable, efficient systems. As a fullstack developer, your goal is to ensure that the data model serves both the backend and frontend needs seamlessly while considering performance, integrity, and scalability. You can become a better fullstack developer by using prompt techniques to complete your tasks for higher quality results at a rapid pace.
Benefits of new style of data modeling - Holistic Programming
- Focus on big picture of system goals - Story & Outcomes
- Instant success and correction control with dynamic code changes
- Dynamic product control by changing story, prompt, AI or manual code changes
- Higher productivity from team and business
- Cost saving on years of error and bug fix cycle
- Efficiency of data based decisions and faster tryouts.
This chapter should provide a solid foundation for understanding and applying data modeling in fullstack development, helping you design more efficient and maintainable applications.
Best
Discover the best practices of building best product experience from millions of ready-made product graphs or build one yourself.

Intelligent
In-depth intelligence of products in the form of product stories help in achieving quality, automation and efficiency in new and existing product implementations.

Augmented
Improve and augment end to end product selection, development, integration, and operation with detailed information and AI copilots.

